The WooCommerce REST API offers robust programmatic access to order data, making it an essential tool for businesses looking to enhance their e-commerce capabilities. Integrating WooCommerce data with external systems becomes crucial in maintaining operational efficiency as your companies expand their online operations.
This guide explains how to manage WooCommerce orders using the REST API with PHP, providing seamless access to your store’s order information. In addition, this integration is especially beneficial for:
- Enterprise system
- CRM Integrations
- Automated workflow management
- Inventory control systems
The capacity to programmatically access and manage order data for growing businesses represents a significant operational advantage. Whether your company requires integration with customer relationship management systems, automated reporting solutions, or sophisticated inventory management tools, the WooCommerce REST API provides the necessary foundation.
In the upcoming sections, we will explore the process of implementing technical features, which includes setting up authentication, using API endpoints, and providing practical code examples. This guide is designed to give you a comprehensive understanding of the WooCommerce Orders REST API and its real-world applications in a business setting.
What is a REST API?
A REST API (Representational State Transfer Application Programming Interface) is a method that allows different applications to communicate with each other over the Internet. REST APIs use standard HTTP with methods (GET, POST, PUT, DELETE) to retrieve or manipulate data in a structured way. In WooCommerce, the REST API enables secure, programmatic access to store data—like products, customers, and orders—making it ideal for integrations and automation.
The REST API allows interaction with different methods. In this case, we will focus only on the GET method to manage orders.
Use case: Integrating WooCommerce orders with a CRM
Let’s explore a scenario where a store owner aims to synchronize WooCommerce orders with a Customer Relationship Management (CRM) system to monitor customer purchases effectively. By utilizing the WooCommerce REST API, the store owner can automatically retrieve orders, enabling the CRM to record order data as it is generated in the WooCommerce store. This integration simplifies the analysis of customer behaviour, allows for tracking of order history, and facilitates sending targeted emails based on customer purchase patterns.
Using the REST API, the store owner can set up an automated script to fetch orders regularly and push them to the CRM, saving time and ensuring accurate, up-to-date data.
Accessing WooCommerce orders through the REST API
- Enable the WooCommerce REST API and generate API Keys.
First, ensure the WooCommerce REST API is enabled and create API keys with the necessary permissions:
- Go to WooCommerce > Settings > Advanced > REST API in your WordPress dashboard.
- Click on Add Key to create a new API key.
- Set the permissions to Read/Write. For this case, only Read is necessary since we will only be retrieving Orders; however, for learning purposes, keep both options.
- Copy the Consumer Key and Consumer Secret for use in your script (Basic Authentication)
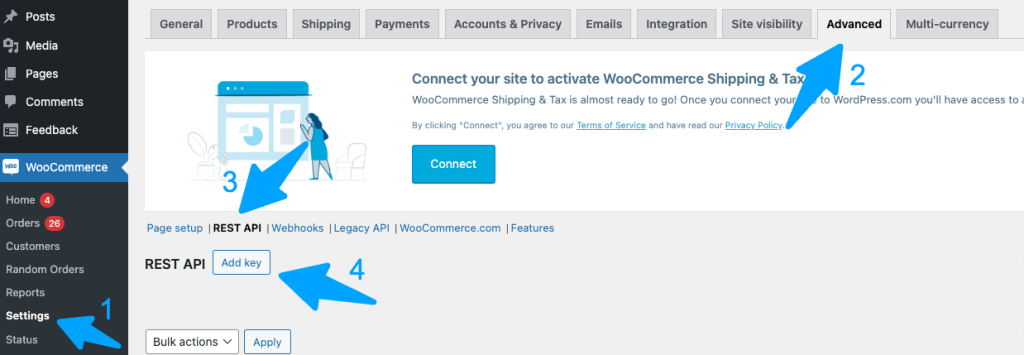
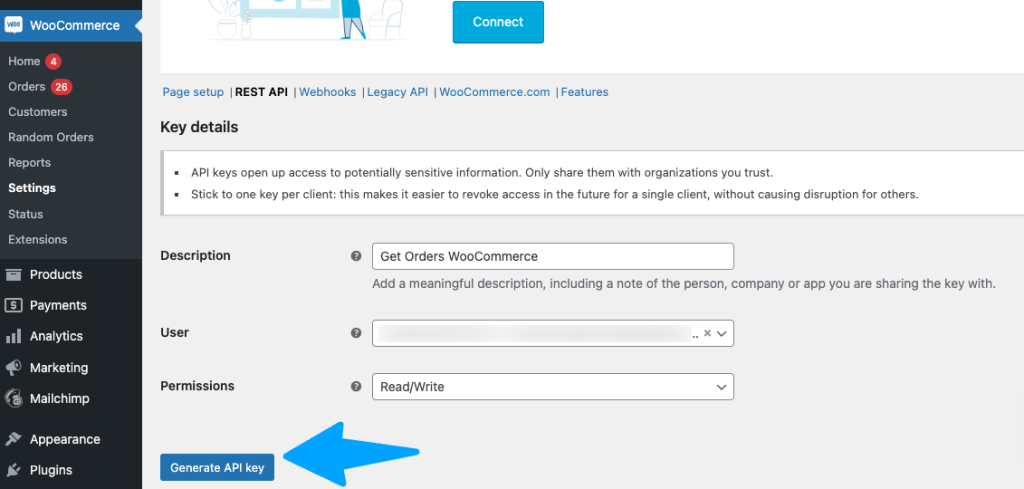
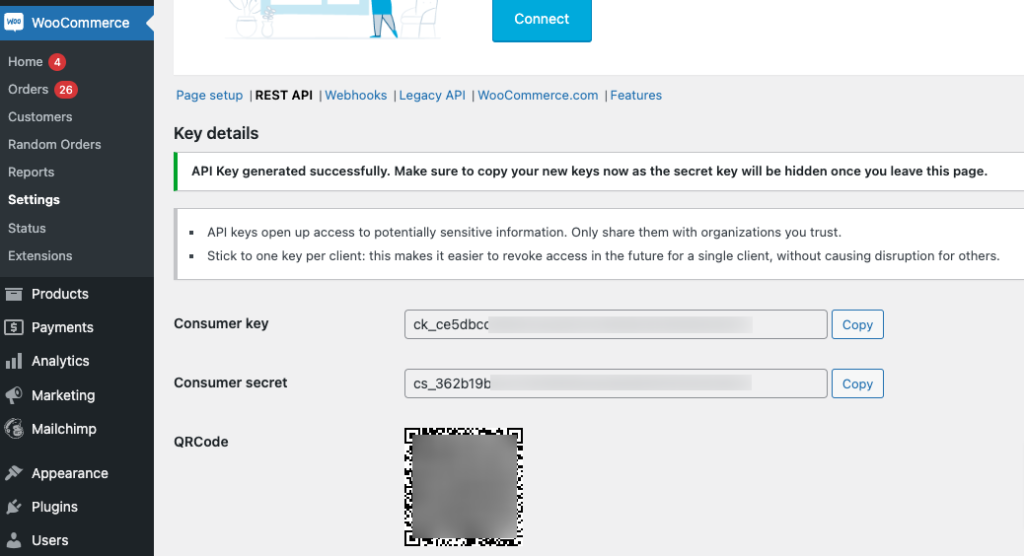
2. Structure the API Endpoint
To begin, it’s important to understand that REST APIs function by using a URL endpoint to retrieve the desired data. In this case, we’re focusing on orders. Below is an example of an endpoint that can be used to fetch orders in WooCommerce. For more detailed information, please refer to the documentation.
https://your-store-url.com/wp-json/wc/v3/orders
Make sure to replace https://your-store-url.com
with the actual URL of your store.
We can use optional parameters to customize the data returned from the URL. I will explain more about this shortly.
?per_page=100
: Sets the number of orders to retrieve per page (up to 100).&page=1
: Specify the page number if you need to paginate through multiple orders.
3. Using Authentication
The WooCommerce REST API uses Basic Authentication, which requires the Consumer Key and Consumer Secret generated in Step 1. For simplicity, we’ll use file_get_contents()
in PHP, but you can also use cURL or a library like Guzzle for more advanced integrations.
PHP script to fetch WooCommerce orders
Below is a PHP script that retrieves all WooCommerce orders by paginating through results. It outputs the order details, including the order ID, status, total amount, date, and the customer’s phone number.
Code Breakdown
- The base URL for accessing WooCommerce orders through the REST API is provided below.
https://your-store-url.com
should be replaced with the actual URL of your WooCommerce store.- The endpoint
/wp-json/wc/v3/orders
indicates the API version (v3
) and the orders resource. There are multiple versions available, but we will use the latest stable one.
// WooCommerce store URL
$store_url = 'https://your-store-url.com/wp-json/wc/v3/orders';
- Consumer Key and Consumer Secret are the credentials you generated in WooCommerce to access the REST API, these keys are used to authenticate API requests and should be replaced with your actual credentials from WooCommerce.
// WooCommerce API credentials
$consumer_key = 'ck_your_consumer_key'; // Replace with your Consumer Key
$consumer_secret = 'cs_your_consumer_secret'; // Replace with your Consumer Secret
$total_orders_to_display
: Specifies the maximum number of orders you want to fetch and display.$per_page
: The number of orders to retrieve per API request, with100
as the maximum WooCommerce allows in one request.
// Configuration variables
$total_orders_to_display = 50; // Set the desired number of orders to display
$per_page = 100; // Maximum allowed by WooCommerce API
- This code sets up Basic Authentication for the request by encoding the Consumer Key and Consumer Secret.
- The
Authorization: Basic
header allows WooCommerce to verify the credentials and grant access to the order data. base64_encode()
securely encodes the credentials so they can be sent in the request.
// Set up authentication using Basic Auth
$options = [
'http' => [
'header' => "Authorization: Basic " . base64_encode("$consumer_key:$consumer_secret"),
'method' => 'GET'
]
];
$page
: Tracks the current page of results being fetched. Pagination starts withpage=1
.$all_orders
: An empty array to store all orders retrieved across multiple pages.
// Initialize variables
$page = 1;
$all_orders = [];
Starting with the pagination loop
- Pagination Loop: This
do...while
loop handles pagination, requesting data until it reaches the specified$total_orders_to_display
. - Constructing the URL:
- Each page URL is formed by appending
?per_page=100
(number of results per page) and&page=$page
(page number). - On each loop iteration,
page
increments by 1 to fetch the next set of orders.
- Each page URL is formed by appending
- Fetching Orders:
file_get_contents($url, false, stream_context_create($options))
sends the API request and retrieves the response, which is stored in$response
.- If
file_get_contents
fails (e.g., due to network or authentication issues), the script will terminate withError fetching orders
.
- Decoding JSON Data:
json_decode($response, true)
converts the JSON response into a PHP array, making it easy to work with the order data.$orders
stores the current page of results.
- Merging Results:
array_merge($all_orders, $orders)
adds the current page’s results to$all_orders
, which accumulates all retrieved orders.
- Loop Condition:
- The loop continues as long as
$orders
is not empty (meaning there are still orders to fetch) andcount($all_orders) < $total_orders_to_display
.
- The loop continues as long as
// Loop through pages to fetch all orders until reaching the desired limit
do {
// Set URL with pagination parameters
$url = $store_url . '?per_page=' . $per_page . '&page=' . $page;
// Fetch the orders from WooCommerce REST API
$response = file_get_contents($url, false, stream_context_create($options));
if ($response === FALSE) {
die('Error fetching orders');
}
// Decode JSON response to PHP array
$orders = json_decode($response, true);
// Merge current page orders with all_orders
$all_orders = array_merge($all_orders, $orders);
// Increase page number for next iteration
$page++;
// Stop fetching more pages if we have reached the desired number of orders
} while (!empty($orders) && count($all_orders) < $total_orders_to_display);
Limiting the orders array
- This line ensures the final array
$all_orders
only contains up to$total_orders_to_display
orders, in case we retrieved extra due to pagination. array_slice($all_orders, 0, $total_orders_to_display)
cuts down the array to the firstn
items.
// Limit the orders array to the specified number of orders
$all_orders = array_slice($all_orders, 0, $total_orders_to_display);
Displaying the orders
- Check for Orders:
if (empty($all_orders))
: This checks if the$all_orders
array is empty, meaning no orders were retrieved. If so, it outputs “No orders found.“
- Output Order Details:
- If orders are retrieved, the script loops through
$all_orders
and outputs details for each order:- Order ID: Unique identifier of the order.
- Status: Current order status (e.g., completed, pending).
- Total: The total amount for the order.
- Date: The date the order was created.
- Phone Number: Customer’s billing phone number, or “N/A” if not available.
- If orders are retrieved, the script loops through
// Check if orders were retrieved
if (empty($all_orders)) {
echo "No orders found.";
} else {
// Display all orders
echo "<h2>WooCommerce Orders:</h2>";
foreach ($all_orders as $order) {
echo "Order ID: " . $order['id'] . "<br>";
echo "Status: " . $order['status'] . "<br>";
echo "Total: $" . $order['total'] . "<br>";
echo "Date: " . $order['date_created'] . "<br>";
// Display the billing phone number
$phone = isset($order['billing']['phone']) ? $order['billing']['phone'] : 'N/A';
echo "Phone Number: " . $phone . "<br><hr>";
}
}
See the entire code below
// WooCommerce store URL
$store_url = 'https://your-store-url.com/wp-json/wc/v3/orders';
// WooCommerce API credentials
$consumer_key = 'ck_your_consumer_key'; // Replace with your Consumer Key
$consumer_secret = 'cs_your_consumer_secret'; // Replace with your Consumer Secret
// Configuration variables
$total_orders_to_display = 50; // Set the desired number of orders to display
$per_page = 100; // Maximum allowed by WooCommerce API
// Set up authentication using Basic Auth
$options = [
'http' => [
'header' => "Authorization: Basic " . base64_encode("$consumer_key:$consumer_secret"),
'method' => 'GET'
]
];
// Initialize variables
$page = 1;
$all_orders = [];
// Loop through pages to fetch all orders until reaching the desired limit
do {
// Set URL with pagination parameters
$url = $store_url . '?per_page=' . $per_page . '&page=' . $page;
// Fetch the orders from WooCommerce REST API
$response = file_get_contents($url, false, stream_context_create($options));
if ($response === FALSE) {
die('Error fetching orders');
}
// Decode JSON response to PHP array
$orders = json_decode($response, true);
// Merge current page orders with all_orders
$all_orders = array_merge($all_orders, $orders);
// Increase page number for next iteration
$page++;
// Stop fetching more pages if we have reached the desired number of orders
} while (!empty($orders) && count($all_orders) < $total_orders_to_display);
// Limit the orders array to the specified number of orders
$all_orders = array_slice($all_orders, 0, $total_orders_to_display);
// Check if orders were retrieved
if (empty($all_orders)) {
echo "No orders found.";
} else {
// Display all orders
echo "<h2>WooCommerce Orders:</h2>";
foreach ($all_orders as $order) {
echo "Order ID: " . $order['id'] . "<br>";
echo "Status: " . $order['status'] . "<br>";
echo "Total: $" . $order['total'] . "<br>";
echo "Date: " . $order['date_created'] . "<br>";
// Display the billing phone number
$phone = isset($order['billing']['phone']) ? $order['billing']['phone'] : 'N/A';
echo "Phone Number: " . $phone . "<br><hr>";
}
}
Review the results of the orders.
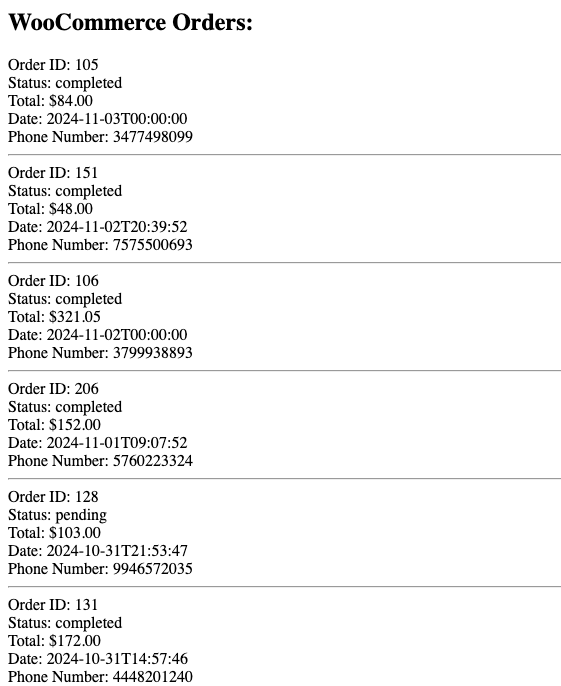
Fetching WooCommerce orders using the REST API allows flexible integration with external applications. By syncing data with a CRM, building custom reporting tools, or integrating with inventory management systems, the WooCommerce REST API allows for programmatic management of order data. You can easily retrieve orders and customize the output for your specific business case with the code provided.
Feel free to ask if you have questions or need advanced customization for WooCommerce API integration!
Leave a Reply